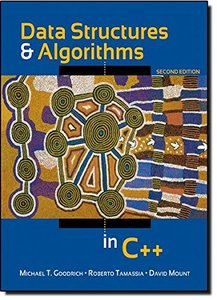
Data Structures and Algorithms in C++, 2/e (Paperback)
內容描述
<內容簡介>
Description
This second edition of Data Structures and Algorithms in C++ is designed to provide an introduction to data structures and algorithms, including their design, analysis, and implementation. The authors offer an introduction to object-oriented design with C++ and design patterns, including the use of class inheritance and generic programming through class and function templates, and retain a consistent object-oriented viewpoint throughout the book.
This is a “sister” book to Goodrich & Tamassia’s Data Structures and Algorithms in Java, but uses C++ as the basis language instead of Java. This C++ version retains the same pedagogical approach and general structure as the Java version so schools that teach data structures in both C++ and Java can share the same core syllabus.
In terms of curricula based on the IEEE/ACM 2001 Computing Curriculum, this book is appropriate for use in the courses CS102 (I/O/B versions), CS103 (I/O/B versions), CS111 (A version), and CS112 (A/I/O/F/H versions).
Features
Consistent object-oriented viewpoint throughout the book.
Detailed explanation and visualization of sorting algorithms.
Coverage of graph algorithms and pattern-matching algorithms for more advanced CS2 courses.
Visual justifications (that is, picture proofs), which make mathematical arguments more understandable for students, appealing to visual learners.
Motivation of algorithmic concepts with Internet-related applications, such as Web browsers and search engines.
Accompanying web site with a special password-protected area for instructors.
New to This Edition
Enhanced consistency with the C++ Standard Template Library (STL), and expanded usage of STL data structures as a basis for designing more complex data structures.
Improved consistency with modern C++ coding standards in presenting code fragments.
Simplification of many of the code fragments, focusing on the principal structure and functionality of the data structures.
More examples and discussion of data structure and algorithm analysis.
Enhanced the discussion of algorithmic design techniques, like dynamic programming and the greedy method.
<章節目錄>
- A C++ Primer.
- Object-Oriented Design.
- Arrays, Linked Lists, and Recursion.
- Analysis Tools.
- Stacks, Queues, and Deques.
- List and Iterator ADTs.
- Trees.
- Heaps and Priority Queues.
- Hash Tables, Maps, and Skip Lists.
- Search Trees.
- Sorting, Sets, and Selection.
- Strings and Dynamic Programming.
- Graph Algorithms.
- Memory Management and B-Trees.